CSS Basic Selectors
Name |
CSS |
Description |
Results |
Universal Selector |
* |
Select all elements |
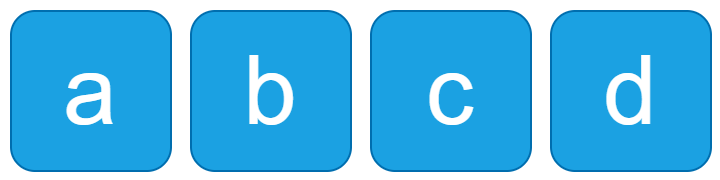 |
Type Selector |
div |
Select elements of that typeSelect div elements |
 |
Class Selector |
.c |
Select elements with that classSelect elements with the c class |
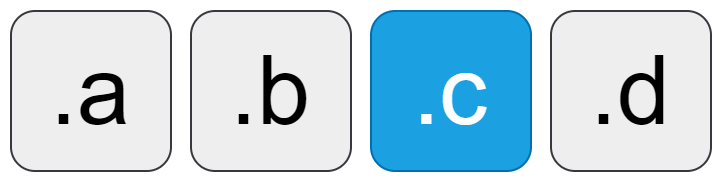 |
ID Selector |
#c |
Select elements with that idSelect elements with the c id |
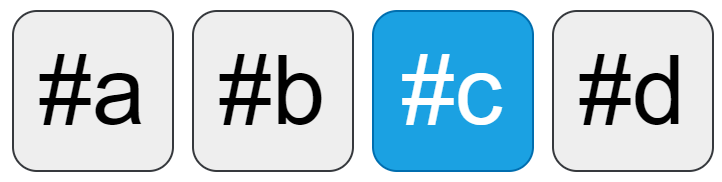 |
CSS Combination Selectors
Name |
CSS |
Description |
Results |
And Selector |
div.c |
Select elements that match all the selector combinations |
 |
Descendant Selector |
div a |
Select elements that are descendants of the first element |
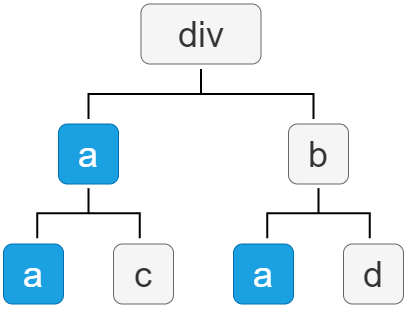 |
Direct Child |
div > a |
Select elements that are direct children of the first element. |
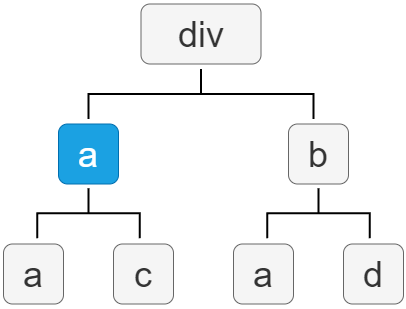 |
Following Sibling |
div ~ a |
Select elements that are siblings of the first element and come after the first element. |
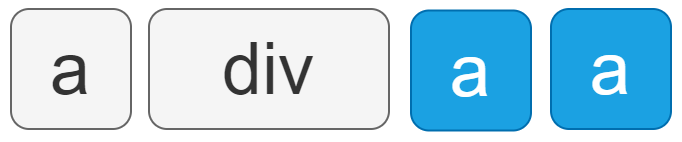 |
Direct Following Sibling |
div + a |
Select elements that are siblings of the first element and come directly after the first element. |
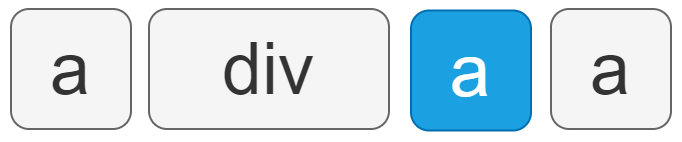 |
Basic CSS Selectors Examples
CSS Type Selector
Type Selector |
div |
Select elements of that typeSelect div elements |
 |
The CSS type selector matches elements by node name. In other words, it selects all elements of the given type within a document.
example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
CSS Class Selector
|
|
|
|
Class Selector |
.c |
Select elements with that classSelect elements with the c class |
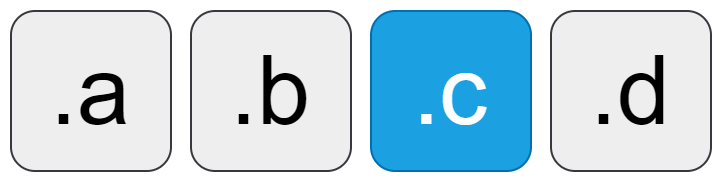 |
The CSS class selector matches elements based on the contents of their class attribute.
example 1
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
example 2
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
CSS Combination Selectors
CSS And Selector
Select elements that match all the selector combinations
|
|
|
|
And Selector |
div.c |
Select elements that match all the selector combinations |
 |
example 1
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
example 2
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
example 3
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
example 4
1
2
3
4
5
6
|
<div class="products">
<p class="product">product 1</p>
<p class="sold product">product 2</p>
<p class="sold product new">product 3</p>
<p class="product-2">product 4</p>
</div>
|
CSS - Direct Child
The > direct child selector selects only direct children of the parent element.
Note that this selector can be dangerous as HTML tree depth can change easily breaking the selector.
example 1:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
example 2:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
Any Descendant Selector
The descendant combinator — typically represented by a single space (" ") character — combines two selectors such that elements matched by the second selector are selected if they have an ancestor (parent, parent's parent, parent's parent's parent, etc.) element matching the first selector. Selectors that utilize a descendant combinator are called descendant selectors.
example 1:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
example 2:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
Any Following Sibling
The following sibling combinator (~, a tilde) separates two selectors and matches all instances of the second element that follow the first element (not necessarily immediately) and share the same parent element.
example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
CSS - Direct Following Sibling
|
|
|
|
Direct Following Sibling |
div + a |
Select elements that are siblings of the first element and come directly after the first element. |
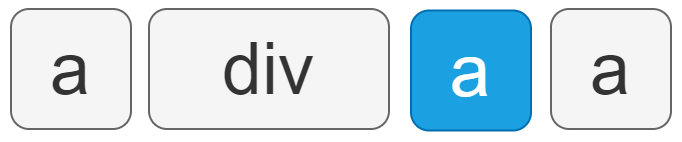 |
Direct Following Sibling (also know as Adjacent Sibling) "+" selects one following adjacent sibling (i.e. has to be right below it). Matches the second element only if it immediately follows the first element, and both are children of the same parent element.
example 1:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<article>
<span>span 1</span>
<p>
Here is a
<span>paragraph
<span>1</span>
</span>
</p>
<code>Here is some html code.</code>
<code>Here is some python code.</code>
<h2>Header with
<span>some pink header</span>
<span>green text</span>
</h2>
<p>Whatever it may be,
<span>keep</span>
<span>smiling.</span>
</p>
<h2>Big
<span>Dream</span>
</h2>
<span>some red text</span>
<span>some blue text</span>
</article>
<span id="span 4">
This span is not selected.
</span>
|
Sources