Python Map, Filter and Reduce functions
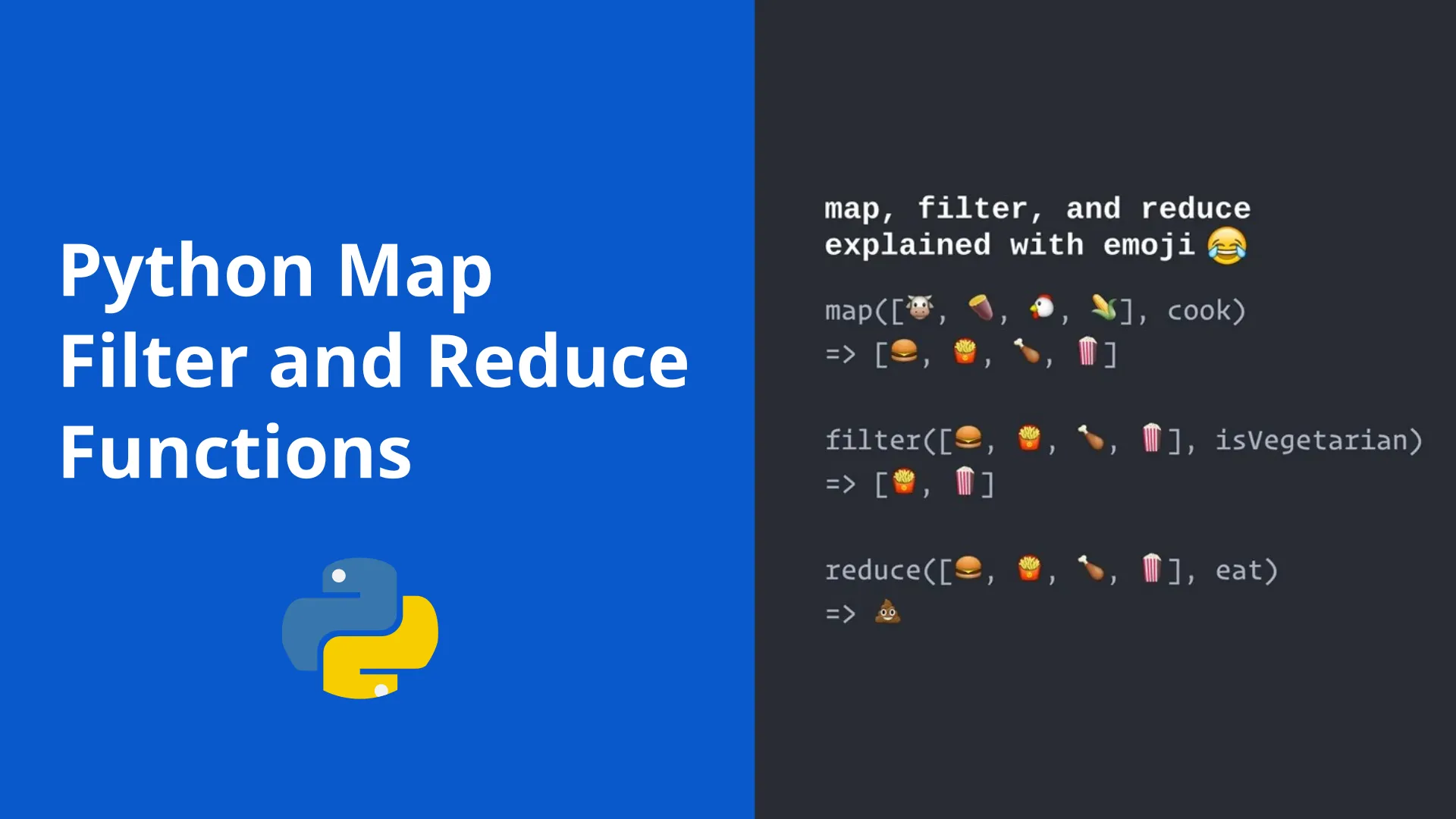
Python Map, Filter and Reduce are three functions which facilitate a functional approach to programming. We will discuss them one by one and understand their use cases.
1. Python Map function
map(function, iterable, ...)
- function - map() passes each item of the
iterable
to this function. - iterable - iterable which is to be mapped
Example 1: Applying a function to all items in one iterable using map()
map(function_to_apply, iterable)
Most of the times we want to pass all the list elements to a function one-by-one and then collect the output. For instance:
items = [1, 2, 3, 4, 5]
squared = []
for i in items:
squared.append(i**2)
print(squared)
# output: [1, 4, 9, 16, 25]
map()
allows us to implement this in a much simpler and nicer way. Here you go:
items = [1, 2, 3, 4, 5]
squared = list(map(lambda x: x**2, items))
print(squared)
# output: [1, 4, 9, 16, 25]
Example 2: Applying a list of function to all items in one iterable using map()
Most of the times we use lambdas with map()
so I did the same. Instead of a list of inputs we can even have a list of functions!
def multiply(x):
return (x*x)
def add(x):
return (x+x)
funcs = [multiply, add]
for i in range(5):
value = list(map(lambda x: x(i), funcs))
print(value)
# Output:
# [0, 0]
# [1, 2]
# [4, 4]
# [9, 6]
# [16, 8]
Example 3: Applying a list constructor to all items to one iterable using the map() function
list('sat')
# Output: ['s', 'a', 't']
words = ['sat', 'bat', 'cat', 'mat']
list(map(list, words))
# Output: [['s', 'a', 't'], ['b', 'a', 't'], ['c', 'a', 't'], ['m', 'a', 't']]
Example 4: Passing more than one iterable to map() function
If additional iterable
arguments are passed, function must take that many arguments and is applied to the items from all iterables in parallel. With multiple iterables, the iterator stops when the shortest iterable is exhausted.
list1 = [4, 5, 6]
list2 = [5, 6, 7]
list(map(lambda n1, n2: n1+n2, list1, list2))
# Output: [9, 11, 13]
list1 = [4, 5, 6, 7, 8, 9]
list2 = [5, 6, 7]
list(map(lambda n1, n2: n1+n2, list1, list2))
# Output: [9, 11, 13]
2. Python Filter function
Python filter function
The filter() function takes a function and a sequence as arguments and returns an iterable, only yielding the items in sequence for which function returns True. If None is passed instead of a function, all the items of the sequence which evaluates to False are removed. The syntax of the filter() is as follows:
filter(function or None, iterable) --> filter object
python filter function example 1
>>>
>>> def is_even(x):
... if x % 2 == 0:
... return True
... else:
... return False
...
>>>
>>> f = filter(is_even, [1, 3, 10, 45, 6, 50])
>>>
>>> f
<filter object at 0x7fcd88d54eb8>
>>>
>>>
>>> for i in f:
... print(i)
...
10
6
50
>>>
To produce the result at once we can use the list()
function.
>>> list(filter(is_even, [1, 3, 10, 45, 6, 50]))
[10, 6, 50]
>>>
>>> list(filter(None, [1, 45, "", 6, 50, 0, {}, False])) # function argument is None
[1, 45, 6, 50]
>>>
python filter function example 2
>>>
>>> filter(lambda x: x % 2 != 0, [1, 3, 10, 45, 6, 50]) # lambda is used in place of a function
[1, 3, 45]
>>>
>>>
>>> list(filter(bool, [10, "", "py"]))
[10, 'py']
>>>
>>>
>>> import os
>>>
>>> list(filter(lambda x: x.startswith(".") != True, os.listdir(".") )) # display all files in the current directory (except the hidden ones)
['Documents', 'Downloads', 'Desktop', 'Pictures', 'bin', 'opt', 'Templates', 'Public', 'Videos', 'Music']
>>>
python filter function example 3
number_list = range(-5, 5)
less_than_zero = list(filter(lambda x: x < 0, number_list))
print(less_than_zero)
# Output: [-5, -4, -3, -2, -1]
The filter resembles a for loop but it is a builtin function and faster.
3. Python Reduce function
The syntax of the reduce() function is as follows:
reduce(function, sequence[, initial]) -> value
The reduce() function accepts a function and a sequence and returns a single value calculated as follows:
- Initially, the function is called with the first two items from the sequence and the result is returned.
- The function is then called again with the result obtained in step 1 and the next value in the sequence. This process keeps repeating until there are items in the sequence. e.g in step 2 it will be 3th item in the sequence, in step 3 it will be 4th item in sequence.
When the initial value is provided, the function is called with the initial value and the first item from the sequence.
from functools import reduce
Here is an example which adds all the items in the list:
from functools import reduce
def do_sum(x1, x2): return x1 + x2
reduce(do_sum, [1, 2, 3, 4])
# output: 10
This reduce()
call perform the following operation:
(((1 + 2) + 3) + 4) => 10
For example, if you wanted to compute the product of a list of integers. So the normal way you might go about doing this task in python is using a basic for loop:
product = 1
list = [1, 2, 3, 4]
for num in list:
product = product * num
# product = 24
Now let’s try it with reduce
:
from functools import reduce
product = reduce((lambda x, y: x * y), [1, 2, 3, 4])
print(product)
# Output: 24
Sources
- http://book.pythontips.com/en/latest/map_filter.html
- https://thepythonguru.com/python-builtin-functions/reduce/