My Python's Ultimate Secrets
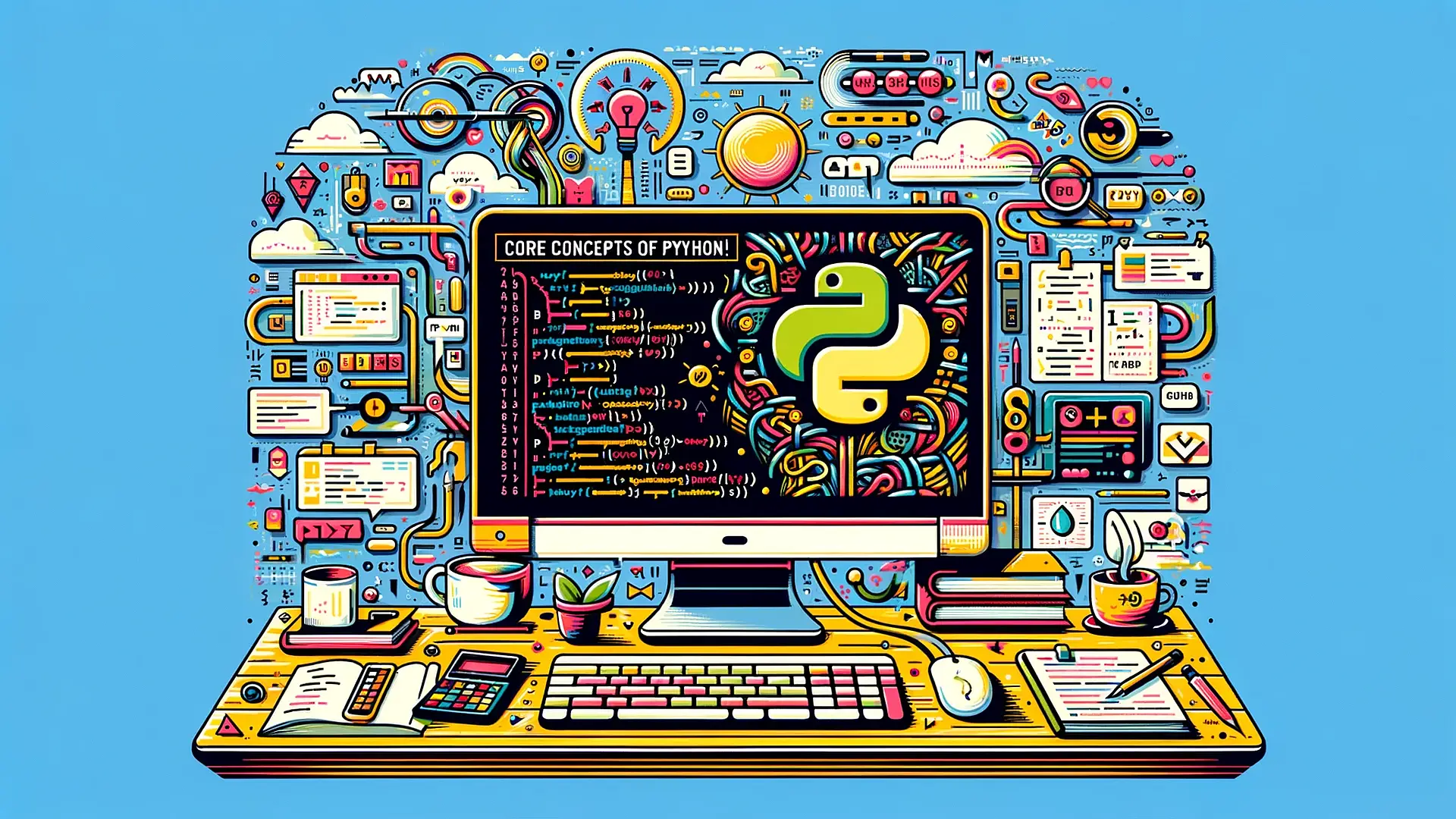
Using __slots__ to reduce memory usage in your classes
In Python, class instances typically harbor their attributes within a __dict__, a built-in dictionary. This feature, while facilitating dynamic attribute assignment, is not without cost—it guzzles memory, especially when instances proliferate. The more instances, the greater the memory overhead, as each one spawns its own __dict__.
Enter __slots__. By defining __slots__ within a class, you explicitly instruct Python to allocate memory for a fixed set of attributes, eschewing the creation of __dict__ altogether. No more dynamic attribute assignment, but what you gain in return is efficiency—a reduction in memory usage that's particularly noticeable in memory-intensive scenarios. Thus, __slots__ becomes a tool of choice when scaling applications, where every byte counts.
Example Usage:
Here's how you can use __slots__ in a Python class:
class Point:
__slots__ = ['x', 'y'] # Only 'x' and 'y' attributes are allowed
def __init__(self, x, y):
self.x = x
self.y = y
# Creating instances of the Point class
p1 = Point(1, 2)
p2 = Point(3, 4)
# Memory usage is reduced since '__dict__' is not created
Benefits
- Memory Efficiency: By not having a __dict__, instances of your class use significantly less memory.
- Speed: Attribute access can be faster because Python doesn't have to look up attributes in a dictionary.
- Immutability of Attributes: Since the attributes are predefined, it's harder (though not impossible) to add new ones dynamically, which can help maintain class integrity.
This trick is particularly useful in scenarios where you are creating large numbers of instances of a class, such as in data processing, simulations, or game development.
Using functools.lru_cache to optimize expensive function calls
The functools.lru_cache decorator—quite the potent tool—lets you stash away the output of a function, so when that function is called again with identical arguments, it simply hands back the cached outcome rather than crunching numbers all over again. Significant boost in performance? Absolutely. Especially when the function in question demands heavy computation or involves operations that drag, like querying databases or similar I/O tasks. Cache it once, and retrieve it fast.
Further reading: pyhon lru cache decorator with time expiration
Using dataclasses with field to customize and optimize data class behavior.
Python 3.7 brought us the gift of dataclasses, a decorator along with functions that magically generate those tedious methods like __init__, __repr__, and __eq__ for you. Boilerplate code? Almost vanished. But here's the twist—hidden within this convenience is a gem not everyone notices: the field() function. It's not just for show; it gives you the reins to tweak, mold, and fine-tune the behavior of individual fields, tailoring each to your whim. Customization, and control — wrapped in a deceptively simple package.
How field() Works
The field() function allows you to specify additional options for each field in a data class, such as default values, default factories, and even metadata that can be used for custom validation or processing.
Example Usage
Here's a simple example that demonstrates how to use python dataclass with field():
from dataclasses import dataclass, field
from typing import List
@dataclass
class Product:
name: str
price: float = 0.0
tags: List[str] = field(default_factory=list) # Use a factory to create a new list for each instance
discount: float = field(default=0.0, metadata={"units": "percentage"}) # Use metadata for additional context
def apply_discount(self):
self.price -= self.price * (self.discount / 100)
# Creating a product
p1 = Product(name="Laptop", price=1000.0, discount=10)
p1.apply_discount()
print(p1) # Output: Product(name='Laptop', price=900.0, tags=[], discount=10.0)
Key Points
- Default Factories: Using default_factory, you can ensure that mutable types like lists or dictionaries are created fresh for each instance, avoiding common pitfalls where multiple instances share the same mutable object.
- Metadata: The metadata parameter allows you to attach custom information to fields, which can be useful for validation, generating user interfaces, or providing context in documentation.
- Customization: field() offers various options, including whether a field should be included in generated methods (init, repr, eq), which provides fine-grained control over how the data class behaves.
- Use Cases: This is particularly useful when you have classes that require dynamic or conditional initialization logic, or when you're building frameworks or libraries where customization and flexibility are key.
Example with Metadata Usage
Here's an example where metadata might be used to add custom validation logic:
from dataclasses import dataclass, field
def validate_positive(instance, attribute, value):
if value < 0:
raise ValueError(f"{attribute} must be positive")
@dataclass
class InventoryItem:
name: str
quantity: int = field(default=0, metadata={"validate": validate_positive})
price: float = field(default=0.0, metadata={"validate": validate_positive})
def __post_init__(self):
for field_name, field_def in self.__dataclass_fields__.items():
validator = field_def.metadata.get("validate", None)
if validator:
validator(self, field_name, getattr(self, field_name))
# This will raise a ValueError due to the negative quantity
item = InventoryItem(name="Widget", quantity=-5, price=10.0)
Using contextlib.suppress to cleanly handle exceptions
contextlib.suppress — a context manager that steps in, silencing specific exceptions within a block of code. No more need for a tangled mess of try-except structures. Cleaner. Leaner. More elegant. It's like telling Python, "Ignore this if it happens," and Python obliges without a second thought. Code stays sharp, clutter fades away. Readability? Uncompromised.
How It Works
When using contextlib.suppress, you specify one or more exceptions that you want to ignore. If any of these exceptions are raised within the with block, they are simply suppressed, and the code continues to execute.
Example Usage
import contextlib
# Example scenario: Trying to delete a file that may or may not exist
import os
filename = 'non_existent_file.txt'
# Traditional approach with try-except
try:
os.remove(filename)
except FileNotFoundError:
pass # Ignore the error if the file doesn't exist
# Cleaner approach with contextlib.suppress
with contextlib.suppress(FileNotFoundError):
os.remove(filename)
Key Points
- Cleaner Code: contextlib.suppress helps you avoid multiple nested try-except blocks, resulting in cleaner and more maintainable code.
- Multiple Exceptions: You can suppress multiple exceptions by passing them as arguments, e.g., contextlib.suppress(FileNotFoundError, PermissionError).
- Use Cases: This is especially useful when you expect certain exceptions to be rare or when the exceptions don't require special handling beyond ignoring them.
Example with Multiple Exceptions
Here's an example that demonstrates how to suppress multiple exceptions:
import contextlib
# Attempting to access and remove files with potential errors
filenames = ['file1.txt', 'file2.txt']
with contextlib.suppress(FileNotFoundError, PermissionError):
for filename in filenames:
os.remove(filename)
In this case, both FileNotFoundError and PermissionError python exceptions will be suppressed, allowing the program to continue without interruption.
Using contextlib.redirect_stdout and contextlib.redirect_stderr to capture output
contextlib.redirect_stdout and contextlib.redirect_stderr — context managers with a knack for diverting the stream. Temporarily hijack where your print statements go, or reroute that standard output and error flow to a file, maybe even a sneaky file-like object. Why? Perhaps to trap output, capture it without lifting a finger to alter the original code. Useful? Absolutely. Especially when third-party libraries are chatty or when debug info pours out like a broken faucet.
How It Works
These context managers? They let you funnel output to virtually any file-like receptacle — be it a simple file, a StringIO, or even the abyss known as /dev/null where output goes to vanish. Perfect when the noise of chatty libraries overwhelms, or when you're keen to capture every line for posterity, stashing it away in a file for post-mortem analysis.
Example Usage
Here's an example of how to capture the standard output into a string using io.StringIO:
import contextlib
import io
# Create a string buffer to capture the output
output_buffer = io.StringIO()
# Redirect stdout to the buffer
with contextlib.redirect_stdout(output_buffer):
print("This will be captured")
print("So will this")
# Retrieve the captured output
captured_output = output_buffer.getvalue()
# Display the captured output
print("Captured output:", captured_output)
Key Points
- Redirect Output: You can redirect both stdout (standard output) and stderr (standard error) to capture or suppress output from your code or third-party libraries.
- File-Like Objects: Redirect to any file-like object, making it highly flexible for logging, testing, or suppressing output.
- Suppress Output: To completely suppress output, you can redirect it to open(os.devnull, 'w').
Example of Suppressing Output
Here's an example of how to suppress output entirely:
import contextlib
import os
# Suppress output by redirecting it to os.devnull
with contextlib.redirect_stdout(open(os.devnull, 'w')):
print("This will not be seen")
print("This will be seen")
Using setdefault to simplify dictionary operations
The setdefault method — a somewhat overlooked yet potent force within Python dictionaries. It's the tool you didn't know you needed, streamlining, simplifying, and slicing through the clutter. What does it do? It quietly checks if a key exists in the dictionary, and if not, it steps in to insert that key with a given value. But it doesn't stop there. It then hands you the value associated with the key, all in one clean, efficient sweep.
How It Works
Typically, when navigating the terrain of dictionaries, you'd toss in an if statement, checking the existence of a key before assigning it a fallback value. Tedious, right? Enter setdefault — where this whole dance condenses into a single, elegant line. Streamlined, sharper, more readable. Code that whispers efficiency, eliminating the need for verbose checks and balances.
Example Usage
Here's a basic example of how to use setdefault:
# Example scenario: counting occurrences of items in a list
items = ['apple', 'banana', 'apple', 'orange', 'banana', 'apple']
# Traditional approach using if-else
counts = {}
for item in items:
if item in counts:
counts[item] += 1
else:
counts[item] = 1
print(counts) # Output: {'apple': 3, 'banana': 2, 'orange': 1}
# Cleaner approach using setdefault
counts = {}
for item in items:
counts.setdefault(item, 0)
counts[item] += 1
print(counts)
# Output:
{'apple': 3, 'banana': 2, 'orange': 1}
Advanced Example with Nested Dictionaries
setdefault becomes even more powerful when dealing with nested dictionaries:
# Example scenario: building a nested dictionary structure
nested_dict = {}
# Traditional approach using if-else
key1, key2 = 'a', 'b'
if key1 not in nested_dict:
nested_dict[key1] = {}
if key2 not in nested_dict[key1]:
nested_dict[key1][key2] = 0
nested_dict[key1][key2] += 1
print(nested_dict) # Output: {'a': {'b': 1}}
# Cleaner approach using setdefault
nested_dict = {}
nested_dict.setdefault(key1, {}).setdefault(key2, 0)
nested_dict[key1][key2] += 1
print(nested_dict)
# Output:
{'a': {'b': 1}}
Using the itertools.groupby function for efficient data grouping
The itertools.groupby function, nestled within Python's itertools module, is a formidable mechanism for clustering sequential elements in an iterable that share a common thread—a key. It doesn't just group; it aligns, categorizes, and organizes. This function excels in scenarios demanding data aggregation, report generation, or the meticulous processing of naturally clustered sequences. It's a tool that, when wielded, transforms scattered data into coherent groups, all by simply sharing a common attribute. Powerful, precise, essential for any data wrangler.
How It Works
The itertools.groupby function? It clusters successive elements in an iterable, each sharing the same value—determined by a specified key function. What it hands you is an iterator of tuples: the first element being the key itself, and the second, a group iterator for elements unified by that key. It doesn't just group; it creates a sequence within a sequence, tying data together based on a common thread, all while keeping it succinct. One function, multiple layers, complex simplicity.
Example Usage
Here's an example that shows how to group data using itertools.groupby:
import itertools
# Sample data: a list of dictionaries representing transactions
transactions = [
{'date': '2024-08-01', 'amount': 100},
{'date': '2024-08-01', 'amount': 150},
{'date': '2024-08-02', 'amount': 200},
{'date': '2024-08-02', 'amount': 50},
{'date': '2024-08-03', 'amount': 300},
]
# Group transactions by date
transactions.sort(key=lambda x: x['date']) # Sort the data by date first
grouped_transactions = itertools.groupby(transactions, key=lambda x: x['date'])
# Process each group
for date, group in grouped_transactions:
print(f"Date: {date}")
for transaction in group:
print(f" Transaction amount: {transaction['amount']}")
# Output:
Date: 2024-08-01
Transaction amount: 100
Transaction amount: 150
Date: 2024-08-02
Transaction amount: 200
Transaction amount: 50
Date: 2024-08-03
Transaction amount: 300
Key Points
- Sorting Required: The input data must be sorted by the key function for groupby to group elements correctly.
- Memory Efficiency: Since groupby returns iterators, it's very memory efficient. It doesn't require loading all the data into memory, making it suitable for processing large datasets.
- Custom Key Functions: You can define a custom key function to group items based on any criteria, providing great flexibility.
Advanced Example: Grouping by a Derived Property
Here's an example of grouping items by a derived property:
import itertools
# Sample data: list of products with categories
products = [
{'name': 'apple', 'category': 'fruit'},
{'name': 'banana', 'category': 'fruit'},
{'name': 'carrot', 'category': 'vegetable'},
{'name': 'broccoli', 'category': 'vegetable'},
{'name': 'cherry', 'category': 'fruit'},
]
# Sort the data by the category
products.sort(key=lambda x: x['category'])
# Group by category
grouped_products = itertools.groupby(products, key=lambda x: x['category'])
# Process each group
for category, group in grouped_products:
print(f"Category: {category}")
for product in group:
print(f" Product: {product['name']}")
# Output:
Category: fruit
Product: apple
Product: banana
Product: cherry
Category: vegetable
Product: carrot
Product: broccoli
The Database Debacle: Inefficient Queries in a Sea of Data
When Simple Queries Became a Bottleneck
One of the more baffling issues we encountered revolved around his approach to database interactions. At Google, where data flows like water through a fire hose, efficiency isn't just a nicety — it's a necessity. Yet, he had this uncanny habit of writing SQL queries directly within loops, turning what should have been a streamlined process into a sluggish, resource-draining operation.
Here's a taste of the chaos:
# His approach
for user in users:
cursor.execute(f"SELECT * FROM orders WHERE user_id = {user.id} AND status = 'complete'")
orders = cursor.fetchall()
for order in orders:
process_order(order)
On the surface, it looks like it does the job. But dig deeper, and you'll realize this is a performance nightmare, especially when dealing with massive datasets. Each iteration triggers a new database query, hammering the database repeatedly, when a single, well-crafted query could have done the heavy lifting.
Now, consider a more optimized alternative:
# A more efficient approach
user_ids = [user.id for user in users]
cursor.execute(f"SELECT * FROM orders WHERE user_id IN ({','.join(map(str, user_ids))}) AND status = 'complete'")
orders = cursor.fetchall()
for order in orders:
process_order(order)
This version? It consolidates the queries into one, drastically reducing the number of database hits. It's leaner. Smarter. This isn't just about speed — it's about treating the database with the respect it deserves, especially when you're working at a scale where inefficiency translates directly into costs.
Yet, somehow, this was a recurring pattern in his code. The kind that makes you wonder how much slower the whole system might have been if this had slipped through unnoticed. The kind of mistake that you wouldn't expect from someone in a leadership role at a place like Google. But, as they say, reality is often stranger than fiction.
Threading Troubles: The Multithreading Misadventure
When Concurrency Became Chaos
In a high-stakes environment like Google, you'd expect mastery over multithreading. After all, handling tasks concurrently should be second nature when working with massive, ever-scaling applications. Yet, here we were, grappling with code that seemed more like a tangle of threads than a finely woven fabric.
The problem? His misguided attempts at managing concurrency with Python's threading module. Instead of using thread pools or asynchronous programming for I/O-bound tasks, he'd launch new threads in a haphazard manner, creating more problems than solutions. The code would spawn threads like wildfire, but there was little to no synchronization, leading to race conditions, deadlocks, and a nightmare of debugging sessions.
# His approach
import threading
for task in tasks:
thread = threading.Thread(target=process_task, args=(task,))
thread.start()
What looks simple is, in reality, a recipe for disaster. Threads were started with reckless abandon, each racing to complete without any form of coordination. No thread joins. No consideration for shared resources. The result? Unpredictable outcomes, with tasks stepping on each other's toes and occasionally bringing the entire system to a grinding halt.
Contrast this with a more methodical approach:
# A more controlled approach
from concurrent.futures import ThreadPoolExecutor
with ThreadPoolExecutor(max_workers=10) as executor:
executor.map(process_task, tasks)
This method not only limits the number of concurrent threads but also ensures they are properly managed, avoiding the pitfalls of unsynchronized execution. It's clean. Predictable. And crucially, it's scalable, which is exactly what you'd expect in a professional setting like Google.
Yet, this wasn't an isolated misstep. His tendency to neglect the nuances of threading led to multiple incidents where we had to firefight unexpected behavior in production. It's the kind of oversight that doesn't just slow things down — it grinds them to a halt, leaving you wondering how such a fundamental concept could be so misunderstood.
Async Apocalypse: Misadventures in Asynchronous Programming
When Concurrency Became a Catastrophe
In the world of professional Python development, especially at a place like Google, you'd expect asynchronous programming to be wielded with precision. It's the tool you reach for when you need to handle thousands, even millions, of I/O-bound operations without breaking a sweat. But in the hands of my team leader, async wasn't just a tool — it was a ticking time bomb, waiting to go off.
The root of the problem? A complete misunderstanding of how to properly use asyncio. Rather than creating non-blocking, efficient code, he managed to create something that was, ironically, slower than its synchronous counterpart. The code was riddled with await calls in places where they did more harm than good, leading to unexpected bottlenecks and unresponsive processes.
# His approach
async def fetch_data(urls):
results = []
for url in urls:
data = await fetch_url(url) # Blocking I/O operation in a loop
results.append(data)
return results
On the surface, this might look like a textbook example of async programming. But dig a little deeper, and it's clear that this approach is fundamentally flawed. By awaiting inside a loop, he inadvertently serialized what should have been a parallel operation, turning a potentially fast, non-blocking process into a sluggish mess. Instead of fetching all URLs concurrently, they were fetched one at a time, defeating the entire purpose of using async in the first place.
Here's how it should have been handled:
# A more effective approach
async def fetch_data(urls):
tasks = [fetch_url(url) for url in urls]
return await asyncio.gather(*tasks) # Fetching all URLs concurrently
This approach, leveraging asyncio.gather , ensures that all the URLs are fetched concurrently, as intended. It's efficient. It's clean. And most importantly, it actually makes use of the asynchronous nature of asyncio to handle multiple tasks at once, reducing the overall runtime significantly.
But somehow, this was a pattern he struggled with repeatedly. Instead of embracing the power of async, he seemed to fight against it, creating more problems than he solved. The irony? His misuse of async often led to worse performance than if he'd just stuck with synchronous code from the start.
Further reading: Mastering Asynchronous Programming in Python: A Comprehensive Guide
Further reading: Asynchronous HTTP Requests in Python with aiohttp and asyncio
Memory Madness: The Case of the Unmanaged Resources
When Memory Leaks Became the Uninvited Guests
In an environment where every millisecond counts and efficiency isn't just preferred but expected, you'd assume memory management would be second nature. But, against all odds, we found ourselves navigating the murky waters of memory leaks — something that should be an anomaly at a place like Google. Yet, here we were, watching as our applications slowly bled resources, all because of a fundamental oversight: failing to release resources in long-running processes.
It wasn't just the fact that objects weren't being properly disposed of; it was the sheer scale of the issue. Consider this piece of code:
# His approach
def process_data(data_list):
results = []
for data in data_list:
results.append(heavy_computation(data))
return results
At first glance, it seems innocent, maybe even trivial. But dig deeper. What happens when data_list is massive, and heavy_computation retains references to large chunks of data? You get bloated memory usage that never quite clears out, leading to a system that eventually suffocates under its own weight. There's no explicit cleanup, no mindful management of the memory footprint, just a slow, creeping disaster.
Now, compare that with a more disciplined approach:
# A more mindful approach
import gc
def process_data(data_list):
results = []
for data in data_list:
result = heavy_computation(data)
results.append(result)
del data # Explicitly delete the reference
gc.collect() # Force garbage collection
return results
In this version, we see an explicit attempt to manage resources — deleting references, forcing garbage collection when necessary. It's not just about keeping memory usage in check; it's about preventing the kind of slow, hard-to-detect leaks that can cripple an application over time. And yet, this was an area where he consistently fell short, opting instead for the "out of sight, out of mind" approach to memory management.
This wasn't a one-off mistake. It was systemic, pervasive, and indicative of a deeper issue: a lack of attention to detail where it mattered most. The kind of mistake that doesn't just cause a minor hiccup but can lead to catastrophic failures in production environments where uptime and reliability are paramount.