Mastering Asynchronous Programming in Python: A Comprehensive Guide
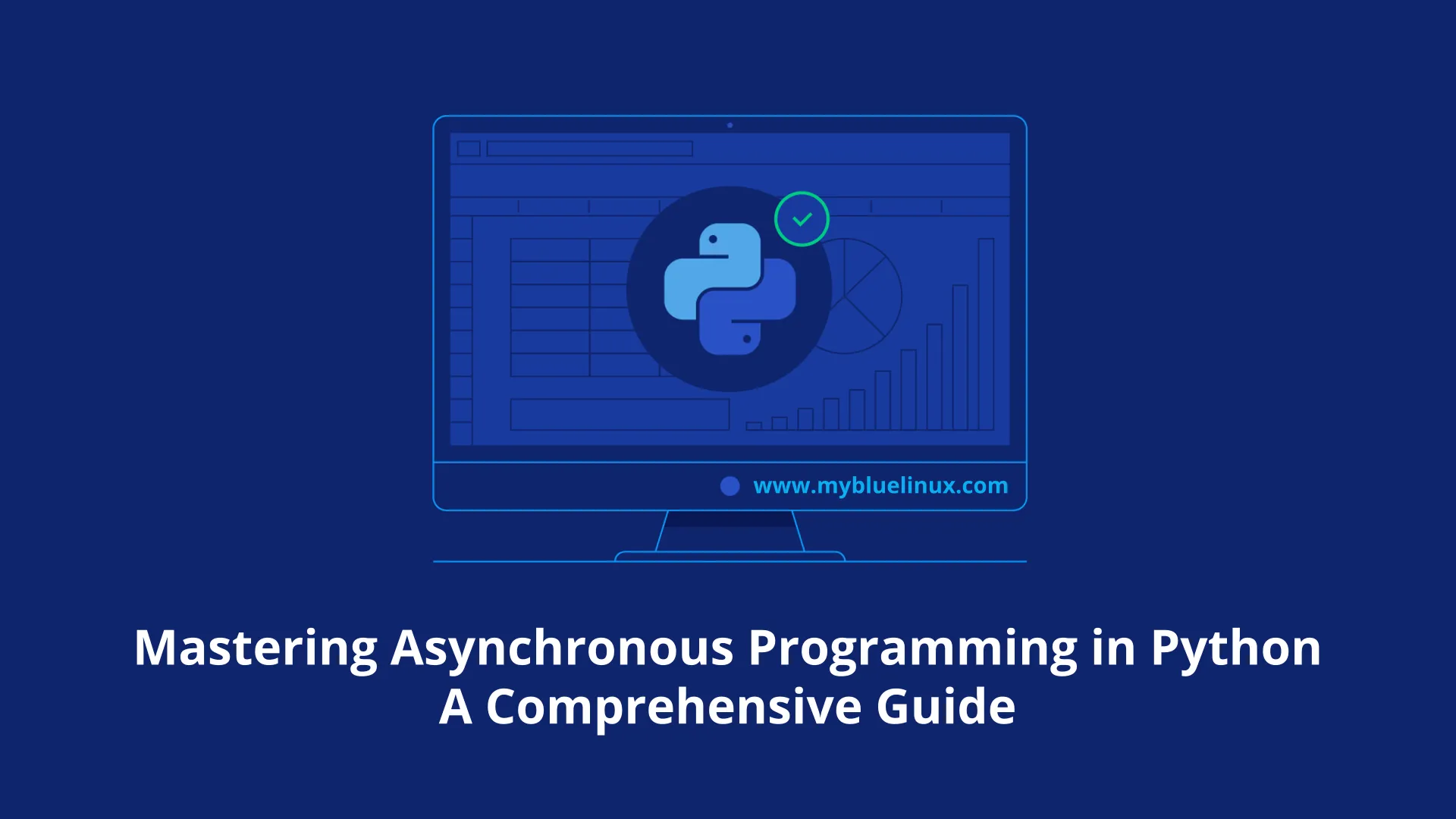
In the ever-evolving landscape of software development, efficiency and performance are paramount. Python, known for its simplicity and readability, has become a preferred language for a wide range of applications. However, as developers push the boundaries of what's possible with Python, the need for more sophisticated programming techniques becomes evident. This is where asynchronous programming, a paradigm that allows for handling multiple tasks concurrently, becomes a game changer.
This comprehensive guide is designed to unravel the complexities of asynchronous programming in Python. Aimed at both beginners and seasoned programmers, it seeks to provide a thorough understanding of the asynchronous programming model and its practical applications in Python.
Understanding the Basics
Before diving into the intricacies of asynchronous programming in Python, it's essential to establish a foundational understanding of what asynchronous programming is and how it contrasts with traditional synchronous programming. This knowledge will not only aid in grasping the more complex aspects of asyncio later on but also in appreciating the nuances of this powerful programming paradigm.
What is Asynchronous Programming?
Asynchronous programming is a paradigm that allows a program to perform multiple operations concurrently. It enables tasks to be initiated and then set aside until results are needed, allowing other tasks to run in the meantime. This approach is especially useful in situations involving waiting for external resources or long-running computations.
Imagine a restaurant kitchen. In synchronous cooking, a chef would complete each task for a dish before starting the next one. In asynchronous cooking, while one dish is in the oven, the chef starts prepping the next one.
Evolution of Asynchronous Programming in Python
Early frameworks like Twisted provided the groundwork for asynchronous programming but were complex and had a steep learning curve. The introduction of generator-based coroutines in Python 3.3 simplified asynchronous programming, leading to asyncio in Python 3.4. The introduction of the async/await syntax in Python 3.5 further enhanced code readability and maintainability, marking a significant step forward in Python's asynchronous programming capabilities.
Synchronous vs. Asynchronous Programming
The main difference between synchronous and asynchronous programming lies in how tasks are executed and managed. Synchronous programming is straightforward but can be inefficient, while asynchronous programming is more complex but significantly more efficient in certain scenarios.
Asynchronous Example:
import asyncio
async def fetch_data():
print("Start fetching")
await asyncio.sleep(2) # Simulating an I/O operation
print("Done fetching")
return {'data': 1}
async def print_numbers():
for i in range(10):
print(i)
await asyncio.sleep(1)
async def main():
task1 = asyncio.create_task(fetch_data())
task2 = asyncio.create_task(print_numbers())
value = await task1
print(value)
asyncio.run(main())
# output:
>>> Start fetching
>>> 0
>>> 1
>>> Done fetching
>>> {'data': 1}
>>> 2
>>> 3
...
>>> 9
This example illustrates basic asynchronous programming in Python using asyncio. Two tasks are defined: fetch_data simulates a delayed I/O operation, and print_numbers prints numbers with a delay. Both tasks are executed concurrently in the main function. Notice how await is used to wait for an operation to complete without blocking the entire program.
Synchronous Example:
# Synchronous code example
import time
def fetch_data():
print("Start fetching")
time.sleep(2) # Simulating a blocking I/O operation
print("Done fetching")
return {'data': 1}
def print_numbers():
for i in range(10):
print(i)
time.sleep(1)
def main():
value = fetch_data()
print(value)
print_numbers()
main()
# output:
>>> Start fetching
>>> Done fetching
>>> {'data': 1}
>>> 0
>>> 1
>>> 2
...
>>> 9
In this synchronous version, the program completes the fetch_data function before starting print_numbers. The sequential nature of the execution is evident as the numbers are printed only after the data fetching is complete.
Best Practices and Considerations
- For I/O-bound operations, asynchronous programming is generally the better choice, as it prevents the program from being blocked while waiting for external operations to complete.
- In CPU-bound tasks, where computations are intensive and continuous, traditional synchronous or multi-threading approaches might be preferable.
- It's essential to manage shared resources carefully in asynchronous programming to avoid race conditions and ensure thread safety.
Corner Cases and Challenges
- Integrating asynchronous code with synchronous libraries can be challenging, as it requires careful management of the event loop.
- Debugging asynchronous code can be more complex due to its non-linear execution flow.
Understanding these core principles of asynchronous programming sets a solid foundation for delving into more complex aspects of asyncio in Python. Recognizing when and how to use asynchronous programming effectively is crucial for Python developers aiming to optimize performance and efficiency in their applications.
Diving into Asyncio
Asyncio is a library in Python that provides a framework for writing concurrent code using the async/await syntax. It is used predominantly for writing single-threaded concurrent programs, ideal for I/O-bound and high-level structured network code.
1. Understanding Event Loop
The event loop is the core of the Asyncio library. It's a programming construct that waits for and dispatches events or messages in a program. In the context of Asyncio, the event loop runs asynchronous tasks and callbacks, performs network IO operations, and runs subprocesses.
The event loop is responsible for managing the execution of asynchronous tasks. It keeps track of all the running tasks and when an operation needs to wait (like an IO wait), it pauses the task and resumes it when the operation can proceed.
How the Event Loop Works
- Running Tasks and Scheduling: The loop executes tasks, which are instances of coroutines that are scheduled to run. When a task awaits on a Future, the loop pauses the task and works on running other tasks.
- Handling IO and System Events: Besides running tasks, the event loop also handles IO and system events. It uses mechanisms like select or poll, provided by the operating system, to monitor multiple streams for activities.
import asyncio
async def main():
print('Hello')
await asyncio.sleep(1)
print('World')
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
loop.close()
In this example, main() is a coroutine. loop.run_until_complete(main()) starts the event loop and runs the main coroutine. The await asyncio.sleep(1) call temporarily pauses the main coroutine, allowing the loop to execute other tasks or handle IO events.
Customizing the Event Loop
Asyncio allows for customization of the event loop behavior. Developers can use different event loop policies to change the default event loop or customize its behavior on different operating systems.
if sys.platform == 'win32':
loop = asyncio.ProactorEventLoop() # for IOCP
asyncio.set_event_loop(loop)
else:
loop = asyncio.SelectorEventLoop() # for Unix
asyncio.set_event_loop(loop)
This example demonstrates setting a different event loop based on the operating system.
Best Practices with Event Loop
- Always use asyncio.run() to run the top-level entry point for your asyncio program. This function creates a new event loop, runs the passed coroutine, and closes the loop.
- Avoid manually creating and managing event loops, unless you have a specific reason. This helps prevent common mistakes like creating multiple event loops in a single-threaded program.
2. Coroutines: The Foundation of Asyncio
Coroutines are the fundamental building blocks of Asyncio. They are special Python functions designed to work with asynchronous operations, defined using async def. Unlike regular functions, coroutines can be paused and resumed, enabling them to handle non-blocking operations effectively.
async def fetch_data():
print("Start fetching")
await asyncio.sleep(2) # Simulates a non-blocking wait
print("Data fetched")
In this example, await asyncio.sleep(2) simulates a non-blocking wait, allowing the event loop to manage other tasks during the 2-second pause.
Note that simply calling a coroutine will not schedule it to be executed:
>>> main()
<coroutine object main at 0x1053bb7c8>
To actually run a coroutine, asyncio provides the following mechanisms:
-
The asyncio.run() function to run the top-level entry point main() function (see the above example.)
-
Awaiting on a coroutine. The following snippet of code will print "hello" after waiting for 1 second, and then print "world" after waiting for another 2 seconds:
import asyncio
import time
async def say_after(delay, what):
await asyncio.sleep(delay)
print(what)
async def main():
print(f"started at {time.strftime('%X')}")
await say_after(1, 'hello')
await say_after(2, 'world')
print(f"finished at {time.strftime('%X')}")
asyncio.run(main())
# expected output:
>>> started at 17:13:52
>>> hello
>>> world
>>> finished at 17:13:55
-
The asyncio.create_task() function to run coroutines concurrently as asyncio Tasks .
-
Let's modify the above example and run two say_after coroutines concurrently:
async def main():
task1 = asyncio.create_task(
say_after(1, 'hello'))
task2 = asyncio.create_task(
say_after(2, 'world'))
print(f"started at {time.strftime('%X')}")
# Wait until both tasks are completed (should take
# around 2 seconds.)
await task1
await task2
print(f"finished at {time.strftime('%X')}")
# Note that expected output now shows that the snippet runs 1 second faster than before:
>>> started at 17:14:32
>>> hello
>>> world
>>> finished at 17:14:34
- The asyncio.TaskGroup class provides a more modern alternative to create_task() . Using this API, the last example becomes:
async def main():
async with asyncio.TaskGroup() as tg:
task1 = tg.create_task(
say_after(1, 'hello'))
task2 = tg.create_task(
say_after(2, 'world'))
print(f"started at {time.strftime('%X')}")
# The await is implicit when the context manager exits.
print(f"finished at {time.strftime('%X')}")
The timing and output should be the same as for the previous version.
3. Tasks: Running Coroutines Concurrently
Tasks in Asyncio are used to schedule coroutines concurrently. When you create a task, it schedules the execution of a coroutine: the event loop can then manage multiple tasks, running them concurrently.
When a coroutine is wrapped into a Task with functions like asyncio.create_task() the coroutine is automatically scheduled to run soon:
import asyncio
async def nested():
return 42
async def main():
# Schedule nested() to run soon concurrently
# with "main()".
task = asyncio.create_task(nested())
# "task" can now be used to cancel "nested()", or
# can simply be awaited to wait until it is complete:
await task
asyncio.run(main())
Behavior and Characteristics
- Concurrency Without Threads: Coroutines and tasks allow for concurrency without the need for traditional threading. This concurrency is achieved through cooperative multitasking, where each task yields control to the event loop at await points.
- Error Handling: Errors in coroutines are handled similarly to regular Python functions. Exceptions can be raised and caught within coroutines. If an exception occurs in a task and is not handled, it is propagated to the task's caller when the task is awaited.
- Cancellation: Tasks can be canceled, which raises an asyncio.CancelledError inside the awaited coroutine. This allows for asynchronous cancellation of operations, a critical feature for responsive applications.
async def main():
task = asyncio.create_task(fetch_data())
await asyncio.sleep(1)
task.cancel()
try:
await task
except asyncio.CancelledError:
print("fetch_data was canceled!")
In this example, the fetch_data task is canceled after 1 second, demonstrating how to cancel tasks and handle the cancellation.
4. Futures: Managing Asynchronous Results
Role of Futures
Futures act as a placeholder for a result that hasn't been computed yet. When a Future object is created, it doesn't have a result. The result is set once the asynchronous task is complete. Futures are often used internally in Asyncio but can also be used directly for more complex asynchronous programming patterns.
Creating and Using Futures
Futures are typically created by Asyncio functions and methods, like loop.create_future() . They provide a way to track when an asynchronous operation is completed and to retrieve its result.
async def main():
loop = asyncio.get_running_loop()
future = loop.create_future()
# Schedule the setting of the result
loop.call_soon(future.set_result, "Future is done!")
result = await future
print(result)
In this example, a future is created and its result is set using call_soon . The await on the future will then wait until the result is available.
Futures vs. Tasks
- Difference: While tasks are used for scheduling and executing coroutines, futures are more general-purpose objects used to represent the result of an asynchronous operation. A task is actually a subclass of a future.
- Usage: Tasks are often more convenient for routine Asyncio programming, as they are specifically designed for coroutines. Futures are more suited for integrating with lower-level asynchronous operations or for interoperability with other asynchronous systems.
Handling Results and Exceptions
- Getting Results: The result of a future is obtained using the result() method. If the future is not done, calling result() will raise an InvalidStateError . If the future has been cancelled, it will raise a CancelledError .
- Error Handling: If the operation encapsulated by the future raises an exception, the future captures that exception. It can be retrieved using the exception() method.
async def main():
future = asyncio.Future()
# Set an exception
future.set_exception(RuntimeError('There was an error'))
try:
result = await future
except RuntimeError as e:
print(f"Caught error: {e}")
asyncio.run(main())
This code demonstrates setting and handling an exception in a future.
5. Streams: Handling Network Operations
Components of Streams:
- Reader: An object representing the readable end of the connection. It provides APIs like read , readline , and readexactly for various reading operations.
- Writer: An object representing the writable end. It offers methods like write and drain to facilitate writing to the connection.
Creating and Using Streams
Asyncio provides asyncio.open_connection() for establishing TCP connections, which returns a reader and a writer object.
async def tcp_echo_client(message):
reader, writer = await asyncio.open_connection(
'127.0.0.1', 8888)
print(f'Send: {message}')
writer.write(message.encode())
data = await reader.read(100)
print(f'Received: {data.decode()}')
print('Close the connection')
writer.close()
await writer.wait_closed()
asyncio.run(tcp_echo_client('Hello World!'))
In this example, a TCP connection to a server running on localhost at port 8888 is established. The message "Hello World!" is sent, and the response is awaited.
Handling Server Side with Stream
asyncio.start_server() is used to start a server. It accepts a client handler coroutine, which is called with reader and writer objects every time a new client connection is established.
async def handle_echo(reader, writer):
data = await reader.read(100)
message = data.decode()
addr = writer.get_extra_info('peername')
print(f"Received {message} from {addr}")
print(f"Send: {message}")
writer.write(data)
await writer.drain()
print("Close the connection")
writer.close()
async def main():
server = await asyncio.start_server(
handle_echo, '127.0.0.1', 8888)
addr = server.sockets[0].getsockname()
print(f'Serving on {addr}')
async with server:
await server.serve_forever()
asyncio.run(main())
Here, the server listens on localhost at port 8888. For each connection, it reads data, prints it, echoes it back, and then closes the connection.
Stream Features and Best Practices:
- Buffering and Flow Control: Streams handle buffering and flow control internally, making it easier to manage large or sporadic data flows.
- Error Handling: Proper error handling in stream operations is crucial. Always ensure to close the connection in case of errors or when the operation is complete.
- SSL/TLS Support: Streams support SSL/TLS out of the box, enabling secure connections with minimal extra configuration.
Advanced Usage of Streams
For more complex scenarios, like handling concurrent connections or implementing custom protocols, consider diving into lower-level constructs like transports and protocols, which offer more control but are more complex to use.
6. Synchronization Primitives: Ensuring Thread Safety
Synchronization primitives are tools that help in coordinating concurrent operations, ensuring that resources are used efficiently and safely. In Asyncio, these are used mainly to prevent data races and ensure thread-safe operations when dealing with shared resources in asynchronous programming.
Common Asyncio Synchronization Primitives
- Lock: A Lock is used to guarantee exclusive access to a resource. Only one coroutine can hold a lock at a time. When a lock is held, any other coroutine trying to acquire it will be paused until the lock is released.
async def locked_task(lock, name):
async with lock:
print(f"{name} has the lock")
await asyncio.sleep(1)
print(f"{name} released the lock")
async def main():
lock = asyncio.Lock()
await asyncio.gather(
locked_task(lock, 'First'),
locked_task(lock, 'Second')
)
asyncio.run(main())
This example demonstrates two tasks attempting to acquire the same lock. The 'First' task acquires it first, and the 'Second' task must wait until the lock is released.
- Event: An Event is used to notify multiple coroutines that some condition has become true. An event object manages an internal flag that can be set to true with the set() method and reset to false with the clear() method.
async def waiter(event):
print('waiting for the event')
await event.wait()
print('event is set')
async def main():
event = asyncio.Event()
waiter_task = asyncio.create_task(waiter(event))
await asyncio.sleep(1)
print('setting the event')
event.set()
await waiter_task
asyncio.run(main())
In this example, the 'waiter' coroutine waits for an event to be set. The event is set after a one-second delay, and the 'waiter' coroutine resumes.
- Semaphore: A Semaphore is used to limit the number of coroutines that can access a particular resource at a time. It's initialized with a counter which decrements when the semaphore is acquired and increments when released.
async def resource_access(semaphore, name):
async with semaphore:
print(f"{name} acquired the semaphore")
await asyncio.sleep(1)
print(f"{name} released the semaphore")
async def main():
semaphore = asyncio.Semaphore(2) # Allow 2 concurrent accesses
await asyncio.gather(
resource_access(semaphore, 'Task 1'),
resource_access(semaphore, 'Task 2'),
resource_access(semaphore, 'Task 3')
)
asyncio.run(main())
This example shows a semaphore allowing two tasks to access a resource concurrently while the third must wait.
Best Practices with Synchronization Primitives
- Avoid Deadlocks: Be cautious of deadlocks, which can occur if coroutines wait on each other in a circular manner. Properly structuring the control flow and avoiding holding locks for extended periods can mitigate this.
- Scoped Locking: Use the async with statement to manage locks, ensuring they are always released, even in case of exceptions.
- Prefer Higher-Level Constructs: Whenever possible, use high-level synchronization primitives provided by Asyncio, as they are designed to work seamlessly with the event loop and coroutines.
7. Advanced Topics in Asyncio
As we delve deeper into the world of Asyncio, we encounter a realm where the basic principles of asynchronous programming intertwine with the more intricate and powerful features of this library. The "Advanced Topics in Asyncio" section is designed for those who have grasped the fundamentals of Asyncio and are ready to explore its more complex capabilities.
Transports and Protocols: Lower-Level Network Handling
Transports and Protocols are core components of Asyncio's networking layer, providing a lower-level interface than streams for handling network communications. They offer more control and flexibility, making them suitable for implementing custom communication protocols and handling complex networking scenarios.
Transports
- Functionality: Transports are responsible for the actual transmission of data. They abstract the details of various types of network communication (TCP, UDP, SSL, etc.) and provide a unified interface for sending and receiving data.
- Custom Transports: While Asyncio provides standard transports, you can also implement custom transports to handle unique network behavior or integrate with different network libraries.
Protocols
- Behavior: Protocols define the application-level behavior of a network connection. They parse incoming data and decide how to respond. This is where you implement your communication logic.
- State Management: Protocols often maintain state information about the connection, which can include things like the amount of data received, the current stage in a communication sequence, or error states.
import asyncio
class EchoProtocol(asyncio.Protocol):
def connection_made(self, transport):
self.transport = transport
def data_received(self, data):
self.transport.write(data) # Echoing back received data
async def main():
loop = asyncio.get_running_loop()
server = await loop.create_server(EchoProtocol, '127.0.0.1', 8888)
await server.serve_forever()
asyncio.run(main())
This example illustrates a basic TCP echo server. When data is received, it is sent back to the client. The protocol handles each connection individually.
Corner Cases and Handling Exceptions
- Connection Management: Properly handle the opening and closing of connections. Ensure that resources are released even in error states.
- Data Boundary Issues: Be aware of data boundary issues in TCP protocols. TCP is a stream-oriented protocol without inherent message boundaries, so your protocol must implement its own message framing.
Best Practices
- Resource Cleanup: Always clean up resources. Make sure transports are closed properly when connections are lost or terminated.
- Error Handling: Implement comprehensive error handling in your protocol methods. Network communication is prone to a variety of issues, such as connection timeouts, data corruption, and disconnections.
- Testing: Thoroughly test your protocol and transport implementations under various network conditions to ensure reliability and robustness.
Advanced Usage
- Custom Protocol Implementation: For specialized needs, such as a proprietary communication protocol, implement your own protocol class, defining how to parse incoming data and respond to it.
- Integrating with External Libraries: For integration with network libraries that don't natively support Asyncio, custom transports can be created to bridge the gap, allowing these libraries to be used within the Asyncio ecosystem.
8. Subprocesses: Working with External Processes
Asyncio provides support for running subprocesses and interacting with them asynchronously. This feature is particularly useful for running external commands, processing their output, and managing their execution within an Asyncio event loop.
Creating and Managing Subprocesses
The primary way to run subprocesses in Asyncio is through the asyncio.create_subprocess_exec and asyncio.create_subprocess_shell functions. These functions are coroutines and return a Process object representing the running subprocess.
async def run_command():
process = await asyncio.create_subprocess_shell(
'echo "Hello World"',
stdout=asyncio.subprocess.PIPE,
stderr=asyncio.subprocess.PIPE)
stdout, stderr = await process.communicate()
print(f"[stdout]\n{stdout.decode()}")
print(f"[stderr]\n{stderr.decode()}")
asyncio.run(run_command())
This example demonstrates running a shell command asynchronously and capturing its output.
Interacting with Subprocesses
- Communicating with a Subprocess: You can interact with the subprocess by reading from and writing to its stdin, stdout, and stderr streams, which are available as asyncio.StreamReader and asyncio.StreamWriter objects.
- Waiting for Completion: The Process object provides methods like wait() to wait for the process to complete and communicate() to send data to stdin and read from stdout and stderr.
Handling Input and Output
- Reading Output: Asynchronously read the output of a subprocess using the stdout attribute of the Process object.
- Sending Input: Write to a subprocess's stdin using the write method of the StreamWriter object associated with it.
Best Practices and Considerations
- Handling Large Outputs: For processes that generate large outputs, it's crucial to continuously read their stdout and stderr to avoid deadlocks.
- Managing Process Lifecycles: Ensure proper management of subprocess lifecycles. Close any pipes and properly terminate processes to avoid resource leaks.
- Security Considerations: When using asyncio.create_subprocess_shell, be cautious of shell injection vulnerabilities. Prefer asyncio.create_subprocess_exec for running known commands.
Advanced Usage
- Custom Process Management: For complex scenarios, you can create custom routines to manage multiple subprocesses, handle their I/O streams, and monitor their statuses concurrently.
- Integrating with Other Asyncio Components: Subprocesses can be integrated with other Asyncio components like queues or events for sophisticated process management and IPC (Inter-process Communication).
9. Queues: Managing Data Flow
Creating and Using Queues
- Queue Creation: An Asyncio queue is created using asyncio.Queue . It can hold a fixed number of items, defined by the maxsize parameter. A queue with maxsize=0 (default) is unbounded.
queue = asyncio.Queue(maxsize=10)
- Adding Items to the Queue: Items are added to the queue using the put coroutine. If the queue is full, put will block until there is free space.
await queue.put(item)
- Removing Items from the Queue: Items are retrieved from the queue using the get coroutine. If the queue is empty, get will block until an item is available.
item = await queue.get()
Producer-Consumer Pattern
- Producer: A producer coroutine puts items into the queue. These items could be data fetched from a network, generated computations, etc.
- Consumer: A consumer coroutine takes items from the queue for processing. Multiple consumer coroutines can be used to process items in parallel.
async def producer(queue):
for i in range(5):
await queue.put(f'item {i}')
await asyncio.sleep(1)
async def consumer(queue):
while True:
item = await queue.get()
print(f'Processed {item}')
queue.task_done()
async def main():
queue = asyncio.Queue()
producers = [asyncio.create_task(producer(queue))]
consumers = [asyncio.create_task(consumer(queue)) for _ in range(2)]
await asyncio.gather(*producers)
await queue.join() # Wait until all items are processed
for c in consumers:
c.cancel()
asyncio.run(main())
Best Practices and Considerations
- Flow Control: Use the maxsize parameter to control the flow of data in your application and prevent the queue from growing indefinitely.
- Task Completion: Use task_done() to indicate that a previously enqueued task is complete. This is particularly important when using queue.join() to wait for all items to be processed.
- Handling Producer-Consumer Termination: Ensure graceful termination of producer and consumer coroutines, especially in long-running applications.
Advanced Usage and Techniques
- Priority Queue: For scenarios where certain items need to be processed before others, asyncio.PriorityQueue can be used.
- LIFO Queue: If a last-in-first-out order is required, asyncio.LifoQueue provides this functionality.
10. Extending Asyncio: Creating Custom Components
Extending Asyncio involves creating custom components like custom event loops, transports, protocols, or utilities that integrate seamlessly with Asyncio's architecture. This allows for tailored solutions that meet specific application requirements not covered by the standard library.
Custom Event Loop
- Purpose: Sometimes, the default event loop may not suit particular needs (like integration with other frameworks or optimization for specific I/O patterns).
- Implementation: Creating a custom event loop involves subclassing asyncio.AbstractEventLoop . You'll need to provide implementations for essential methods like run_forever , run_until_complete , and the scheduling and handling of tasks.
# This is a conceptual example. Actual implementation details may vary.
class CustomEventLoop(asyncio.AbstractEventLoop):
def run_forever(self):
while True:
events = external_event_check() # Hypothetical external event check
for event in events:
self._process_event(event)
# Usage
loop = CustomEventLoop()
asyncio.set_event_loop(loop)
Here, CustomEventLoop integrates an external event system into Asyncio's event loop. This might be necessary for applications that rely on a non-standard event source.
Custom Executors
- Purpose: Executors in Asyncio allow the execution of synchronous code in separate threads or processes, enabling non-blocking integration with synchronous libraries or long-running tasks.
- Customization: You might need a specialized executor to manage how synchronous tasks are run, perhaps to integrate with a specific thread or process management system. By implementing custom executors, you can define precisely how these tasks should be handled, scheduled, and executed.
import concurrent.futures
class CustomExecutor(concurrent.futures.ThreadPoolExecutor):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
# Additional initialization here
# Custom behavior can be implemented here
# Usage
executor = CustomExecutor()
asyncio.get_event_loop().run_in_executor(executor, some_blocking_io_task)
This CustomExecutor extends the standard thread pool executor, allowing for customized thread management, which could be tailored for specific types of blocking I/O tasks.
Creating Custom Transports and Protocols
- Custom Transports: While Asyncio provides standard transports for TCP, UDP, and other protocols, you might encounter scenarios where you need a specialized transport layer. For instance, integrating with a non-standard networking library or hardware. In such cases, you can create custom transport classes derived from asyncio.BaseTransport .
- Custom Protocols: Similarly, if the existing protocol abstractions don't fit your needs, you can define custom protocol classes. This might be necessary for implementing a proprietary communication protocol or handling data in a specific format.
class CustomProtocol(asyncio.Protocol):
def connection_made(self, transport):
self.transport = transport
print("Custom Protocol Connection Made")
def data_received(self, data):
print(f"Data received: {data.decode()}")
self.transport.write(b"Echo: " + data)
async def main():
loop = asyncio.get_running_loop()
server = await loop.create_server(
lambda: CustomProtocol(),
'127.0.0.1', 8888)
async with server:
await server.serve_forever()
asyncio.run(main())
This example demonstrates a basic custom protocol echoing received data. This protocol could be further extended to handle more complex data processing.
Best Practices for Extending Asyncio
- Ensure Compatibility: When extending Asyncio, maintain compatibility with its asynchronous model. Custom components should not block the event loop.
- Robust Testing: Extensively test custom components under various conditions to ensure they work reliably with Asyncio's ecosystem.
- Performance Considerations: Be mindful of the performance implications of custom extensions, especially in the context of high-load scenarios.
Conclusion
In this article, we've navigated the multifaceted world of Asyncio in Python, exploring its key features from basic concepts to advanced functionalities. We began with the essentials of asynchronous programming and moved through core Asyncio components like the event loop, coroutines, tasks, and futures. We then progressed to more complex topics, including streams for network operations, synchronization primitives, and advanced areas like custom transports, protocols, and subprocess management.
The journey into Asyncio's depths reveals its power and versatility in handling concurrent operations, making it an invaluable tool for modern Python developers. As Asyncio continues to evolve, it offers an ever-expanding landscape for efficient and scalable application development.
Keep experimenting and leveraging the Asyncio library in your Python projects, and join the vibrant community for ongoing learning and growth in this exciting area of asynchronous programming.