Understanding Django ORM Foreign Key Deletion Constraints
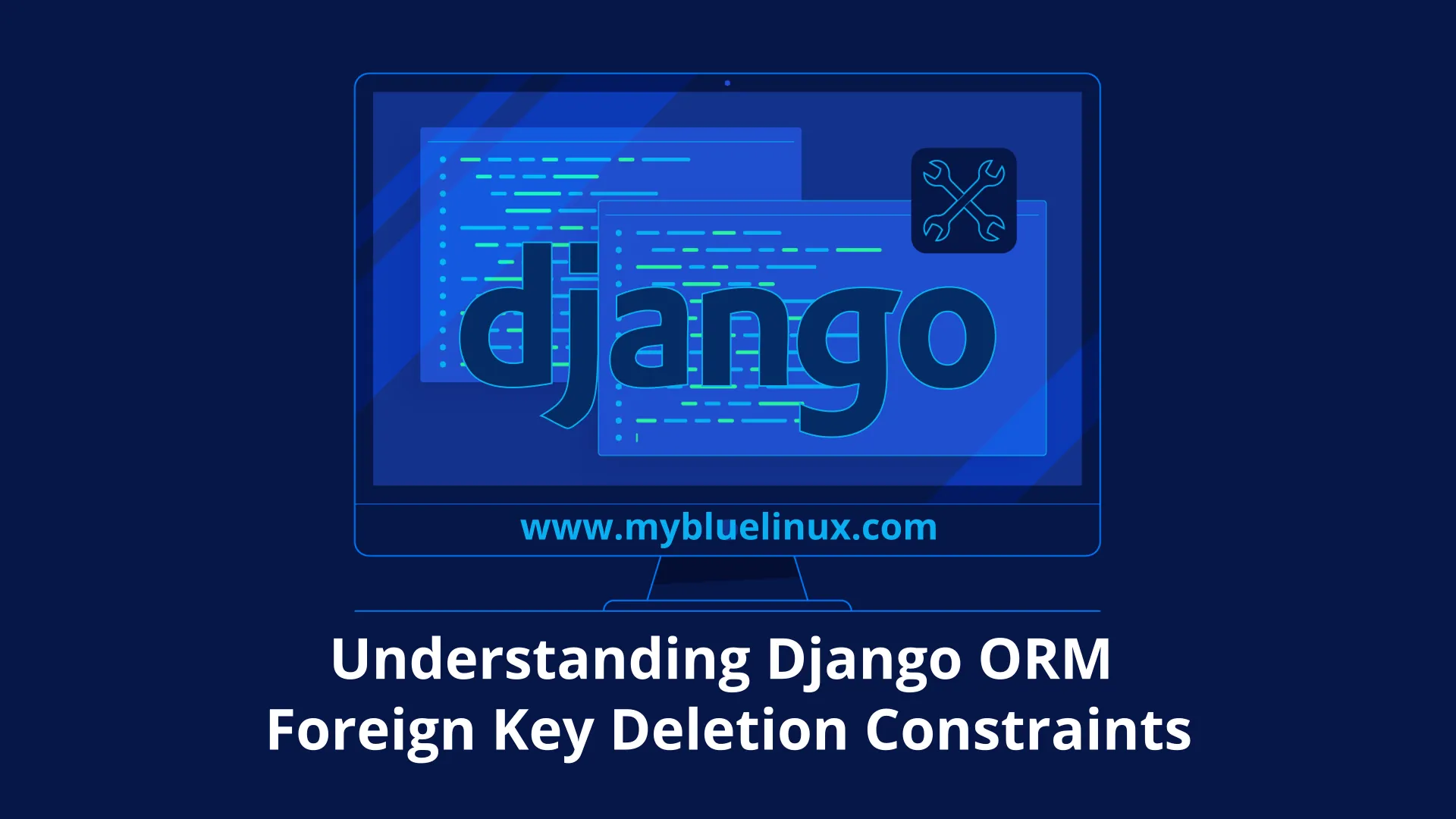
Django, a high-level web framework for Python, provides a powerful Object-Relational Mapping (ORM) system that simplifies database interactions. One essential feature of Django’s ORM is the ability to define relationships between models using Foreign Keys. While Foreign Keys facilitate data integrity and consistency, it’s crucial to understand and manage the deletion of related objects to avoid unintended consequences. In this article, we’ll explore Django ORM Foreign Key deletion constraints and how they impact your database.
The Basics of Foreign Keys in Django
In Django models, a Foreign Key is a field used to establish a many-to-one relationship between two models. The model containing the Foreign Key is referred to as the “source” model, while the model being pointed to is the "target" model. The Foreign Key field in the source model creates a link between instances of the source and target models, establishing a relationship between them.
Here’s a simple example:
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
In this example, the Book model has a Foreign Key author pointing to the Author model. The on_delete=models.CASCADE
parameter indicates that if an Author instance is deleted, all associated Book instances will be deleted as well.
Deletion Constraints: Protecting Data Integrity
The on_delete
parameter is crucial for specifying the behavior when a referenced object is deleted. Django provides several options, each representing a different deletion constraint. Let's explore some of the common ones:
CASCADE
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.CASCADE)
- Behavior: When the referenced Author is deleted, all associated Book instances will be deleted as well.
- Use Case: Use
CASCADE
when a child object’s existence depends on the parent, and it should be removed when the parent is deleted.
PROTECT
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.PROTECT)
- Behavior: Prevents deletion of the referenced Author if there are associated Book instances. An exception will be raised.
- Use Case: Use
PROTECT
when you want to ensure that the referenced object cannot be deleted as long as there are dependent objects.
SET_NULL
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.SET_NULL, null=True)
- Behavior: When the referenced Author is deleted, the author field in associated Book instances is set to NULL (assuming the field allows null values).
- Use Case: Use
SET_NULL
when you want to retain the Book instances but nullify the reference to the deleted Author.
SET_DEFAULT
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.SET_DEFAULT, default=default_author_id)
- Behavior: Similar to SET_NULL, but the author field is set to its default value instead of NULL.
- Use Case: Use
SET_DEFAULT
when you want to assign a default value to the ForeignKey field when the referenced object is deleted.
SET()
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.SET(get_default_author))
- Behavior: Allows you to specify a function (in this case, get_default_author) to determine the value to set on the ForeignKey field.
- Use Case: Use
SET()
when you need custom logic to determine the replacement value for the ForeignKey field.
DO_NOTHING
class Book(models.Model):
# ...
author = models.ForeignKey(Author, on_delete=models.DO_NOTHING)
- Behavior: Does nothing when the referenced Author is deleted. It's up to you to handle the situation manually (e.g., through database triggers or application logic).
- Use Case: Use
DO_NOTHING
when you want to handle the deletion manually, outside of Django’s automatic cascade.
Choosing the Right Deletion Constraint
The choice of deletion constraint depends on the specific requirements of your application and the desired behavior when referenced objects are deleted. Consider the following factors:
-
Data Integrity: Choose a deletion constraint that aligns with your data integrity requirements. CASCADE and PROTECT are often used to enforce strict integrity, while SET_NULL and SET_DEFAULT provide more flexibility.
-
Business Logic: Consider the business logic of your application. For example, if deleting an author should also delete their books, CASCADE is appropriate. If you want to nullify the author field in books, SET_NULL may be a better choice.
-
Default Values: If you have default values for fields, SET_DEFAULT might be a suitable option. It allows you to replace the deleted reference with a predefined default.
-
Custom Logic: If you need custom logic to handle the situation when a referenced object is deleted, SET() or DO_NOTHING may be more appropriate.
Best Practices
- Document Your Choices: Clearly document the deletion constraints in your models. This helps other developers understand the intended behavior and prevents accidental data loss.
- Test Deletion Scenarios: Test your deletion scenarios thoroughly, especially if you choose CASCADE or any other option that involves automatic deletion. Ensure that your models behave as expected and that data integrity is maintained.
- Consider Database Triggers: In some cases, database triggers might be necessary to handle cascading actions or custom logic that Django’s deletion constraints can’t cover.
- Think Long-Term: Consider the long-term implications of your deletion constraints. Changing constraints later may be complex if your application is already in production.
Conclusion
Django’s ORM Foreign Key deletion constraints are a powerful tool for managing relationships between models and ensuring data integrity. By carefully choosing the appropriate deletion constraint for each relationship, you can define the desired behavior when referenced objects are deleted. Whether it’s cascading deletions, protecting against deletions, or custom handling, Django provides a flexible and robust system to suit various application needs. Always consider the specific requirements of your application and choose the deletion constraint that aligns with your data integrity and business logic goals.